GUIs
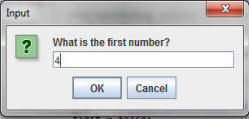
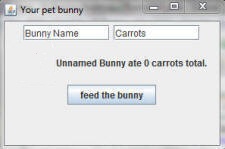
GUI_IO.java - input and output message boxes
Outer.java - buttons and actionlisteners
MakeText.java - text boxes
Bunny.java
BunnyGUI.java
Read D&D 12, 22
Classwork
(This time we will hand code the GUI -- it is okay if your GUI
design is not beautiful.)
Create a class Account which has instance variables for the name of the account owner and the current balance.
Create a class BankGUI that inherits from JFrame. Also have a
main that just creates an instance of BankGUI, to start things off.
Give BankGUI an instance variable of type Account.
You can do most of your setup in the BankGUI constructor, or put it in other
methods called by the constructor. Start off the account with a
default name and a balance of 100.
Add two
labels for the name and balance and set them based on the values from the
Account. Remember that you need to set them up, add them to the BankGUI,
and do setVisible(true) at the end of the constructor, to get anything
to come out.
Of the actionlisteners you will now write, at least one must be an inner class
(non-anonymous) and at least one must be an anonymous inner class.
Add a button labeled Quick Cash. Set up its actionlistener so that when the user clicks the button,
we withdraw $20
from the Account's balance only if the balance is at least that high
(remember to update the display
label to show the new balance). If the balance is not high enough, leave the balance alone and pop up an output message
(using JOptionPane) to complain.
Add an editable text field, which the user can use to enter an amount of money
to be deposited. Set up its actionlistener so that when the user hits enter from this,
we deposit the given amount into the Account Don't forget to update the
display label too. If the input given is just not valid (e.g. not a number), pop up a message box telling the user so.
EC +10
Start the program from a main by popping up an input box
(using JOptionPane) to ask the user for a name, and pass this to the BankGUI
constructor to use when it sets up the Account object.
EC+10 Add another text field for a custom withdrawal amount, which works
like the deposit field, but also, if the amount given to withdraw is larger than the balance, set balance to 0 and pop up an output message to complain.