07A Inheritance
& Polymorphism
Monster.java
VampireBat.java
MuppetMonster.java
MonsterTestHarness.java
Monster
MuppetMonster
VampireBat
Test Harness
Inheritance notes
Polymorphism notes
Read chapter 9-10.
Homework
A zoo is developing an educational safari game with various animals. You will write classes representing Elephants, Camels, and Zebras. 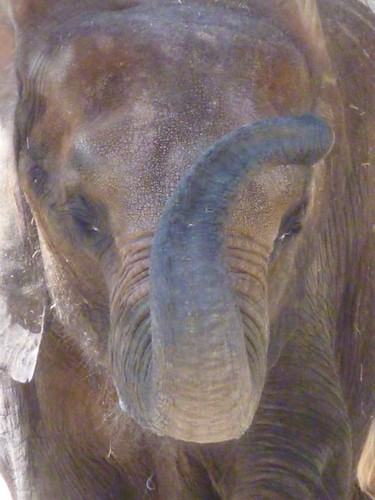
Part A
Think through common characteristics of animals, and write a class ZooAnimal in a package zoo.
ZooAnimal should have at least two instance variables of different types (protected),
representing characteristics that all animals have values for. It should
also have at least two methods for behaviors that all animals do; these can simply print out a
String stating what the animal is doing. (accessors and mutators are not behaviors).
Include at least one parameterized constructor and a toString as well
(no need to include the superclass version of toString unless you think it is
appropriate).
Part B
Create classes Elephant, Camel, and Zebra, all of which extend ZooAnimal (and
are in the same package). In each, include a default constructor that calls the
parameterized superclass constructor.
To one class (of Elephant, Camel, and Zebra), add another instance variable
specific to that type of animal. To another, add another behavior
method. In a third, override a behavior from ZooAnimal.
Part C
Create a test harness for the animal classes. In a main create an array of
ZooAnimals and, and populate it so it has some elements from each class.
Loop through, calling the behavior methods from ZooAnimal for all the elements.
For at least one type, this should result in the overriding method being called.
In the loop, use instanceof to detect animals of whichever type adds a method,
and call that method for that type.
EC [+15] Create another animal class inheriting from one of your three types,
and either add an instance variable or add or override a method. This class should also be tested in the harness.