GUIs
GUIs
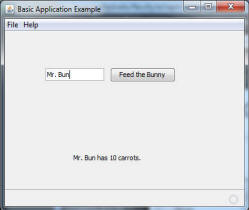
Read ch 14, 15
Classwork
Part A
Create a new Java project and add a class Account which has instance variables for the name of the account owner and the current balance.
Add a new JFrame form to the project.
To the GUI, add a JLabel and in Properties, under Code, rename the variable
for the JLabel to output.
Go to the source code for the GUI and add an instance variable of
type Account. You don't have to worry about accessors, mutators,
etc, in user interface classes
At the end of the GUI class's
automatically generated constructor, set up the account instance var with a name and
an initial balance of $100. Also set the text of the output JLabel
to show information about the account, e.g. "Account owner
Constance de Coverlet has $5003.00" Do this by pulling the values
from the account instance variables, not by keeping extra variables in
the GUI class!! (hint: think about using Account's toString).
Run the program and make sure it is showing the right output.
Part B
Add a button, set its text to Deposit, and set its variable name to
depositButton. Add an actionPerformed Handler for the button
(remember you can do this by double-clicking the button). Doing this should take you to a new method
generated by the design view. In this method, add code to deposit $20 in the Account (that
is, increase the current balance by 20) and update the output label
(that is, set its text) to show the new values in the Account.
[EC]
Add another button, set its text to Quick Cash, and its variable name to
withdrawButton. When the user clicks the button, withdraw $20 only if the balance is at least that high and update the
label accordingly. If the balance is not high enough leave the balance alone.